Integrate DeepSeek AI into React Native app: Full guide for Generative AI in React Native
Learn how to integrate DeepSeek's powerful AI capabilities into React Native applications, with code examples and best practices for implementation.
Posted by

Related reading
What Apple's iOS 18 SDK Update Means for React Native Developers: A Practical Guide
Learn how Apple's iOS 18 SDK requirements affect React Native developers and what steps to take to ensure your apps remain compliant by April 2025.
React Native Open Source Projects Scaling to Millions of Users
Explore the top open-source React Native projects of 2025 and learn how they're scaling to serve millions of users.
Free React Native Templates for 2025: Reduce the Time to Market
Discover the best free React Native templates for 2025. These templates are designed to help you build modern, responsive mobile apps quickly and efficiently.
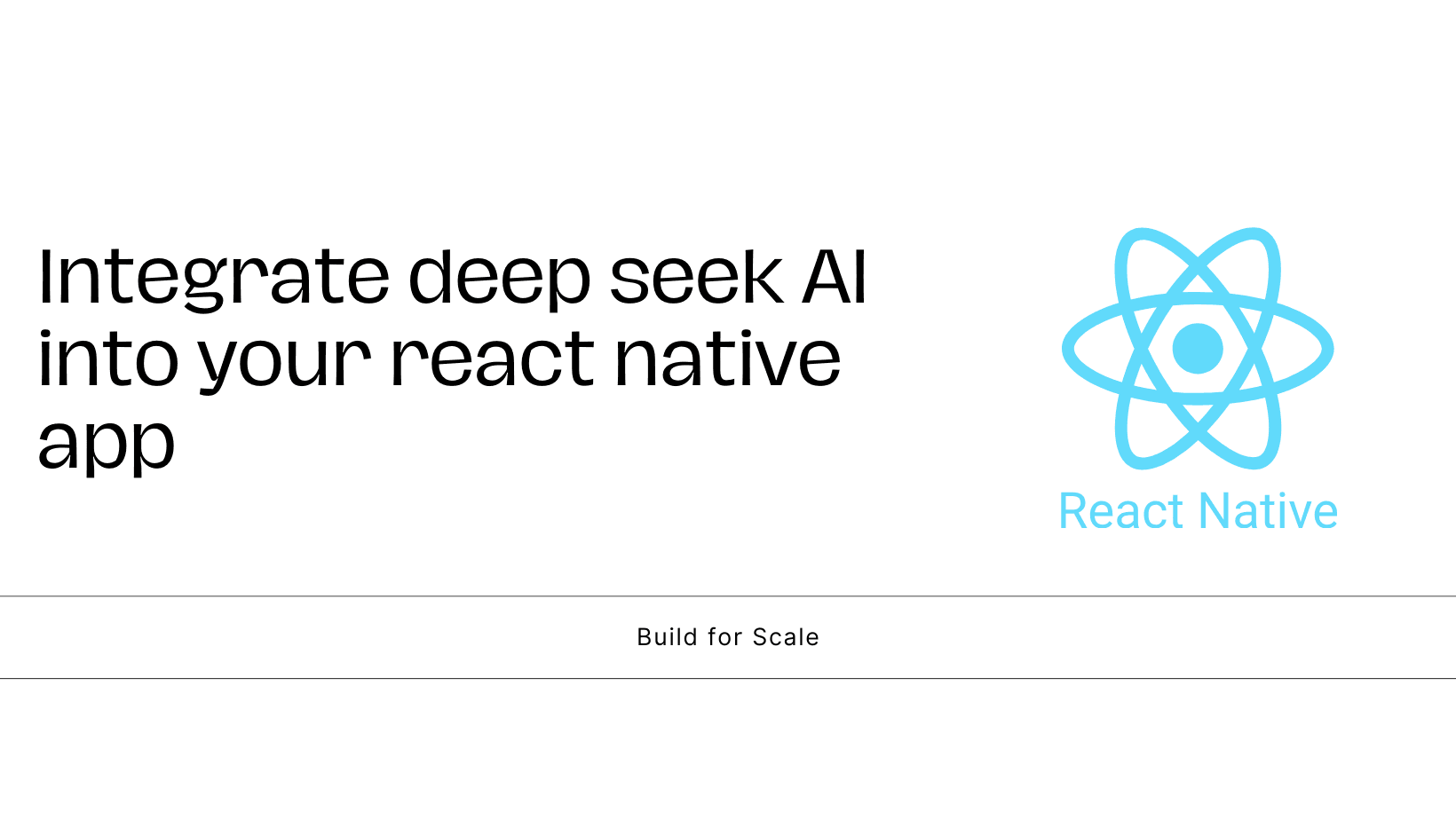
Introduction
React Native has revolutionized mobile app development by enabling developers to build native applications using JavaScript and React. Since its introduction by Facebook (now Meta) in 2015, it has become one of the most popular frameworks for cross-platform mobile development, powering thousands of apps across iOS and Android platforms.
We've witnessed an unprecedented race in generative AI development in the last year. While OpenAI's GPT series and Anthropic's Claude have dominated headlines, open-source alternatives like Meta's Llama and DeepSeek are rapidly gaining traction. These open-source models are particularly interesting because they offer comparable performance while providing more flexibility and control over deployment.
Companies are racing to create an AGI that can solve all the humans problems, although the road is still far for that in my opinion, but we are getting closer each time, and billions of dollars are spent on that. For example the 500 billions projects in USA and the 50 billions AI investment from UAE in France.
DeepSeek, in particular, has shown impressive results in recent benchmarks. According to their technical report, DeepSeek has demonstrated superior performance in coding tasks and mathematical reasoning compared to other open-source models. Their 67B parameter model has achieved competitive results against GPT-4 in several benchmarks, making it an attractive option for developers looking for powerful AI capabilities in their applications.
DeepSeek's impressive performance in benchmarks suggests a promising future for open-source AI models. As these models continue to improve, developers will have more options for implementing AI capabilities in their applications without being locked into proprietary solutions.
The ability to self-host these models or use them through APIs provides flexibility in deployment options, which is particularly important for applications with specific privacy or regulatory requirements.
In this article, I will explain to you how to use it inside a React and React Native app, although the funny part is that they use Open ai SDK package to integrate their solution, which is a clever move from the company to make the switch easy, as the price/performance quote is much better if you use Deepseek that using Open AI models
Integrating DeepSeek AI in React Native
Let's walk through the process of integrating DeepSeek AI into a React Native application to create an AI chat interface.
1. Setting Up the Project
First, ensure you have the necessary dependencies installed:
npm install react-native-gifted-chat axios react-native-paper react-native-safe-area-context // or if you are using Yarn yarn install react-native-gifted-chat axios react-native-paper react-native-safe-area-context
2. Creating the Chat Interface
The implementation uses react-native-gifted-chat, a popular library for building chat interfaces in React Native. Here's how to structure your component:
import React, {"{useState, useCallback, useEffect}"} from 'react'; import {"{GiftedChat, IMessage}"} from 'react-native-gifted-chat'; import axios from 'axios';
3. Configuration
Set up your DeepSeek API credentials:
const DEEPSEEK_API_URL = 'https://api.deepseek.com/v1/chat/completions'; const DEEPSEEK_API_KEY = 'Your_API_Key_Here';
4. Implementing the Chat Logic
The core functionality revolves around two main parts:
Message Management
const [messages, setMessages] = useState<IMessage[]>([]); const [loading, setIsLoading] = useState(false); // Initialize with a welcome message useEffect(() => {'{'} setMessages([ {'{'} _id: 0, text: 'Type your question or share what's on your mind…', createdAt: new Date(), user: {'{'} _id: 0, name: 'DeepSeek', // url for deepseek logo avatar: 'https://cdn.deepseek.com/platform/favicon.png', {'}'}, {'}'}, ]); {'}'}, []);
6. API Integration
const sendMessageToDeepSeek = async (userMessage: IMessage) => { setIsLoading(true); try { const response = await axios.post( DEEPSEEK_API_URL, { model: 'deepseek-chat', messages: [{role: 'user', content: userMessage}], }, { headers: { Authorization:Bearer {DEEPSEEK_API_KEY};, Authorization:, 'Content-Type': 'application/json', }, }, ); // Process response and update chat } catch (error) { console.error('DeepSeek API Error:', error); } finally { setIsLoading(false); } };
7. UI Customization
const renderInputToolbar = (props: InputToolbarProps<IMessage>) => ( <InputToolbar {...props} containerStyle={{backgroundColor: '#f0f0f0'}} /> ); const renderSend = (props: SendProps<IMessage>) => ( <Send {...props}> <View style={styles.sendButton}> <Icon source="send" color="blue" size={30} /> </View> </Send> ); return ( <GiftedChat messages={messages} onSend={messages => onSend(messages)} onInputTextChanged={setText} bottomOffset={insets.bottom} renderSend={renderSend} renderInputToolbar={renderInputToolbar} renderChatFooter={renderFooter} scrollToBottomComponent={renderScrollToBottom} user={{ _id: '1', name: 'Malik', avatar: PROFILE_IMAGE, }} /> )
The interface can be customized using custom renderers:
Check the full projects and code here:
React Native Template
React Native Template, contains beautifully designed, expert crafted React Native components and screens. To Help you…
https://reactnativetemplates.com/screensCode/19Best Practices and Considerations
- API Key Security: Never expose your API key in the client-side code. Use environment variables or a backend service to secure your credentials.
- Error Handling: Implement robust error handling for API calls and network issues.
- Loading States: Provide visual feedback during API calls using loading indicators.
- Message Persistence: Consider implementing local storage to persist chat history.
Ideas and Discussion
The recent surge in Generative AI and AI agents seems heavily focused on web and desktop platforms. While some mobile apps offer wrappers for services like ChatGPT or, like Apple Intelligence, integrate AI directly, the future of AI in mobile app development remains unclear.
One theory suggests lightweight, mobile-friendly AI models are the answer. Meta's work on a package for running AI models locally in React Native (I will write an article to bring more details about it later) supports this idea.
Others believe Generative AI wrappers will drive app development, but I question their revenue potential. These apps often rely on the same underlying model's users can access directly. For example:
- Consider calorie tracking: I can achieve this with ChatGPT, likely at a lower cost since I'm already using ChatGPT for other tasks. Why pay for a dedicated app when a broader platform can handle it?
Other suggests a hybrid approach, with lightweight AI models locally, and heavy computation sent through API
The landscape is still evolving. I'd love to hear your thoughts and predictions on the future of AI in mobile. Share your assumptions and ideas in the comments below.
Conclusion
Integrating DeepSeek AI into a React Native application is straightforward and opens up possibilities for creating sophisticated AI-powered chat interfaces. As the AI landscape continues to evolve, having knowledge of implementing these integrations becomes increasingly valuable for modern mobile developers.
This article provides a comprehensive overview of integrating DeepSeek AI with React Native, from the technical implementation details to the broader industry context. You may want to add specific benchmark numbers and more recent comparisons as they become available, as well as any additional implementation details specific to your use case.
Get the Best React Native Templates in 2025: Community work
Jumpstart your app development with high-quality React Native templates! Discover a variety of pre-designed screens and code examples at React Native Templates:
https://reactnativetemplates.com/
Want to contribute and showcase your React Native expertise? You can easily add your own templates to share with the community and gain valuable exposure. Browse our diverse collection of React Native screens to find the ideal foundation for your next project.
If you need to integrate Advanced functionalities in your Mobile app, create one from scratch, or need consulting in react native. Visit the casainnov.com, and check their mobile app page, and contact them there.